Plugins
1. Introduction
The first objective of BaseReader was to allow any programmer to create easily his own plugin.
As described before, BaseReader without plugin will be unable to read any file.
In this chapter I'll create from scratch a simple plugin to open a plain text file (.txt).
2. How to
2.1 Create your project
First of all, download the latest version of BaseReader.
We will need the "BaseReaderPluginInterfaces.dll".
Note: you can download the sources and add the project "BaseReaderPluginInterfaces.csproj" to your solution. But for this tutorial, I will explain using the already compiled interface.
Open your favorite IDE. In this exemple, I'll use Visual Studio 2010.
Create a New Project > Visual C# > Windows.
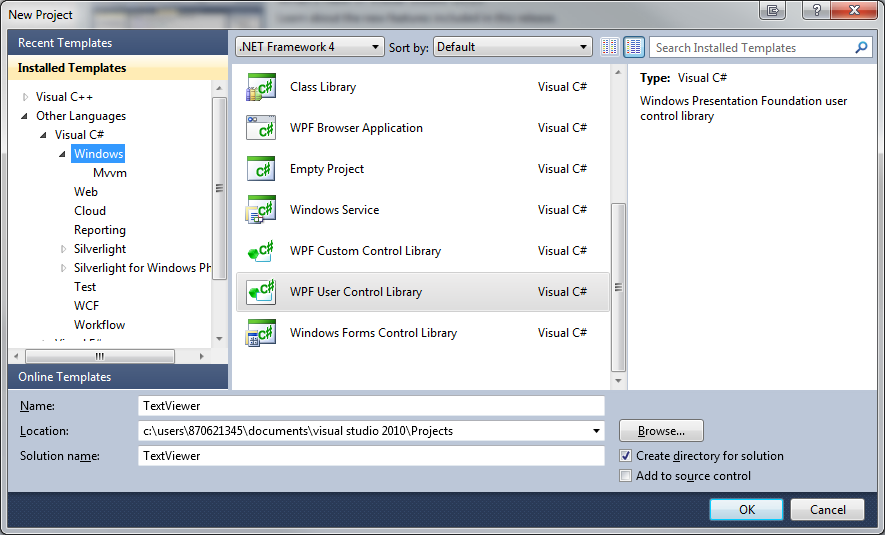
Choose WPF User Control Library (.NET Framework 4)
Name it "TextViewer".
We will need the "BaseReaderPluginInterfaces.dll".
Note: you can download the sources and add the project "BaseReaderPluginInterfaces.csproj" to your solution. But for this tutorial, I will explain using the already compiled interface.
Open your favorite IDE. In this exemple, I'll use Visual Studio 2010.
Create a New Project > Visual C# > Windows.
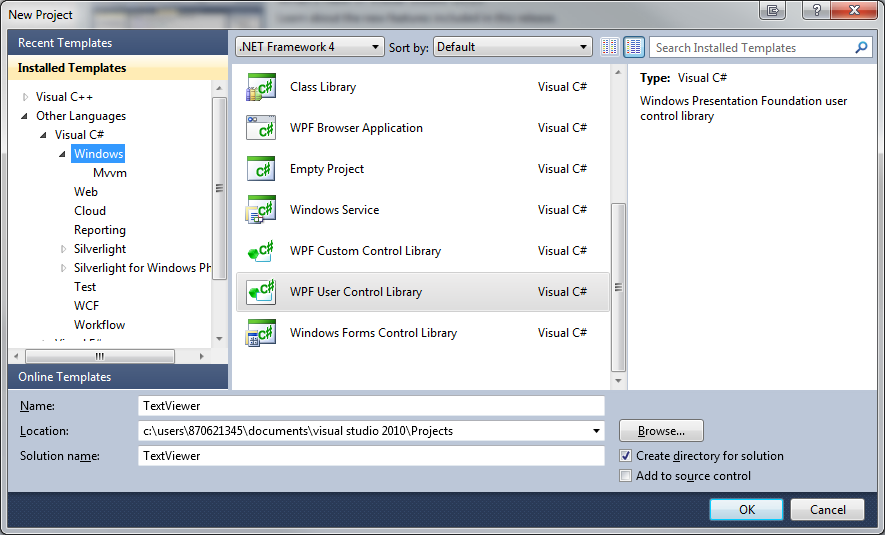
Choose WPF User Control Library (.NET Framework 4)
Name it "TextViewer".
2.2 Setup your project
Now add a reference to "BaseReaderPluginInterfaces.dll" in your project.
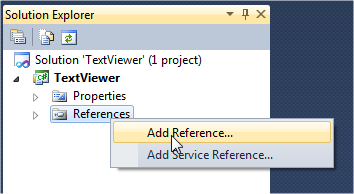
You can browse it to discover the contained interface classes.
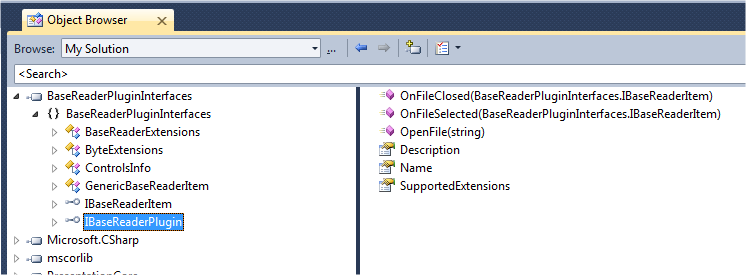
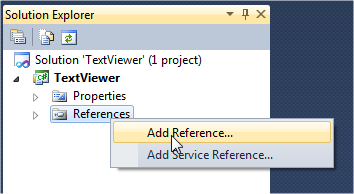
You can browse it to discover the contained interface classes.
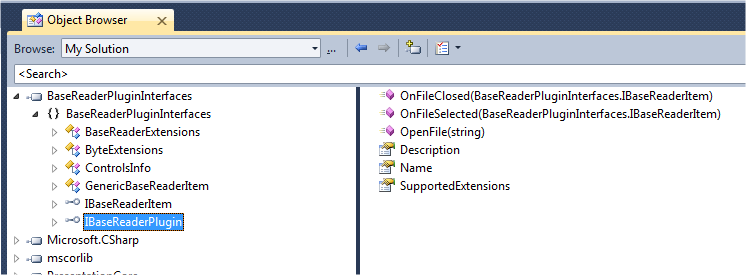
2.3 Create your plugin
Add a new class name "TextReader.cs" that will inherit from "IBaseReaderPlugin".
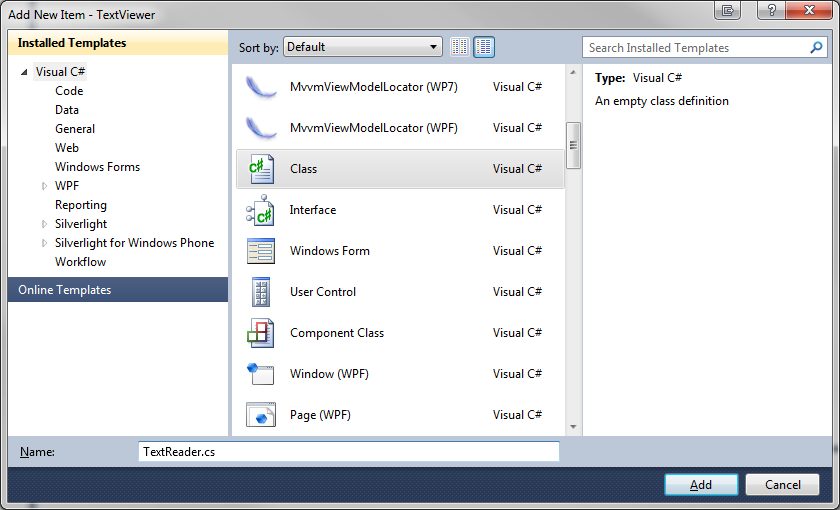
Inherit from IBaseReaderPlugin then create the Properties based on the interface contract.
This assembly provides the class "GenericBaseReaderItem" that inherit from "IBaseReaderItem"
You can use it to display standard controls, and/or do simple viewer.
Now declare the function OpenFile to open the file (provided by BaseReader) then read its content:
Note: You don't have to check the given File Path, BaseReader had previously checked if the file exists, and if the extension is supported.
Declare the remaining methods/properties (from IBaseReaderPlugin)
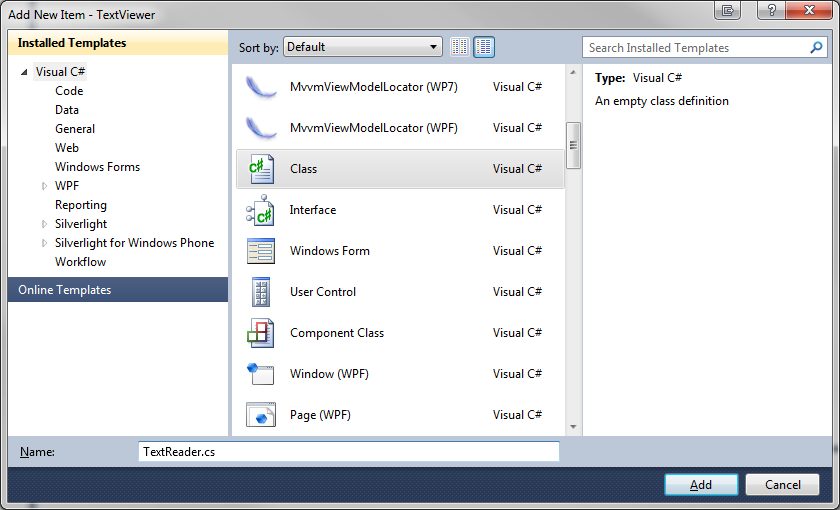
public interface IBaseReaderPlugin { string Description { get; } string Name { get; } string[] SupportedExtensions { get; } void OnFileClosed(IBaseReaderItem _SelectedItem); void OnFileSelected(IBaseReaderItem _SelectedItem); IBaseReaderItem OpenFile(string _FilePath); }
Inherit from IBaseReaderPlugin then create the Properties based on the interface contract.
public class TextReader : IBaseReaderPlugin { private String[] m_SupportedExt = new String[] { ".txt" }; public String[] SupportedExtensions { get { return m_SupportedExt; } } public String Name { get { return "TextReader"; } } public String Description { get { return "Plain Text format reader"; } }
This assembly provides the class "GenericBaseReaderItem" that inherit from "IBaseReaderItem"
You can use it to display standard controls, and/or do simple viewer.
Now declare the function OpenFile to open the file (provided by BaseReader) then read its content:
public IBaseReaderItem OpenFile(String _FilePath) { GenericBaseReaderItem elem = new GenericBaseReaderItem(); var tb = new System.Windows.Controls.TextBox(); using (StreamReader sr = new StreamReader(_FilePath)) { tb.Text = sr.ReadToEnd(); } elem.ViewItem = tb; return elem; }
Note: You don't have to check the given File Path, BaseReader had previously checked if the file exists, and if the extension is supported.
Declare the remaining methods/properties (from IBaseReaderPlugin)
public void OnFileClosed(IBaseReaderItem _SelectedItem) { } public void OnFileSelected(IBaseReaderItem _SelectedItem) { }
2.4 Test your plugin
Build your project, you are done!
You can now copy your plugin in the "Plugins" folder by the BaseReader.exe application file.
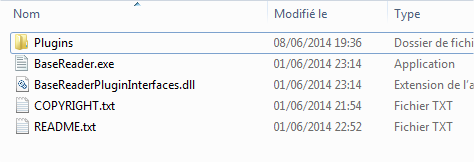
Note: you can set a post-build script to easily tests your plugin.
Once done, run BaseReady, your plugin should be loaded:
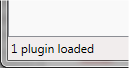
You can drag-n-drop any text file on BaseReader...
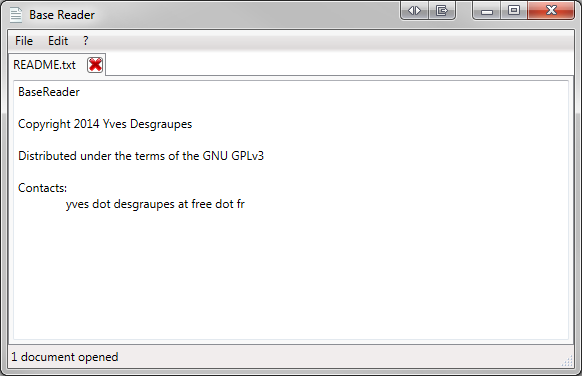
You can dowload the sources of the TextViewer here: TextViewer Sources - VS2010
You can now copy your plugin in the "Plugins" folder by the BaseReader.exe application file.
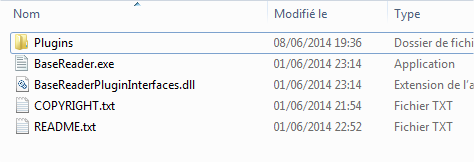
Note: you can set a post-build script to easily tests your plugin.
Once done, run BaseReady, your plugin should be loaded:
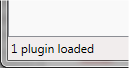
You can drag-n-drop any text file on BaseReader...
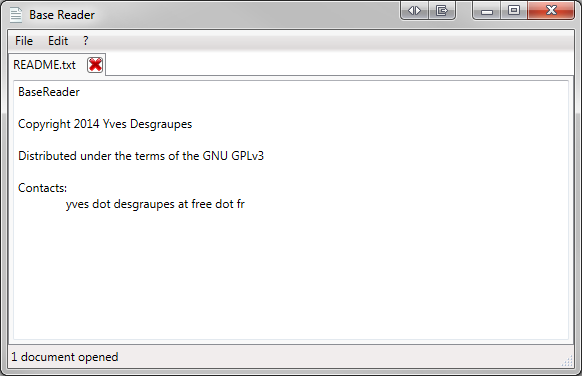
You can dowload the sources of the TextViewer here: TextViewer Sources - VS2010